How To Send A Post Request Using Node.js
By Nicholas Brown.
If you are building a web app that needs to send data to an API, you can do so using an HTTP POST request. Some RPC APIs require you to send a POST request -- even if you are trying to retrieve data from them. The example in this article uses the Axios library to send a POST request to another server in Node.js.
The first step to sending your POST request is to obtain the credentials required for your API. This is not always required, but it may be if you are accessing a third-party API. In this example, we will use another Node.js server running on the same machine (127.0.0.1/localhost).
Create a Node.js app and name it 'test.js', then add the Axios module to it as shown on the first line of code below. Also run 'npm install axios' in the same directory as your app. You can use 'npm install --save' if you want Axios to be installed automatically if you delete the downloaded Axios module or move the app to a machine that does not have it.
Example:
const axios = require('axios');
//Let us submit a simple request for contact message in the form of a JSON object.
let jsondata = {"Name": "Nicholas Brown", "Email:" : "name@emailprovider.com", "Message": "Hi, I would like to discuss the 'Starter' advertising package for my company. When can we arrange a Jitsi call?"};
axios.post('http://127.0.0.1:8080/contact', { jsondata })
.then(function (response) {
console.log(response); //Print the server's response to the console.
})
.catch(function (error) {
console.log(error); //Display errors thrown, if any.
});
There are several security considerations to be made. However, this example is merely a bite-sized starting point that I kept short for the sake of simplicity.
The 'let jsondata=...' line creates a JSON object with some dummy data to send in your POST request. The code that follows starting 'axios.post' constructs the POST request and sends it off to the server/API.
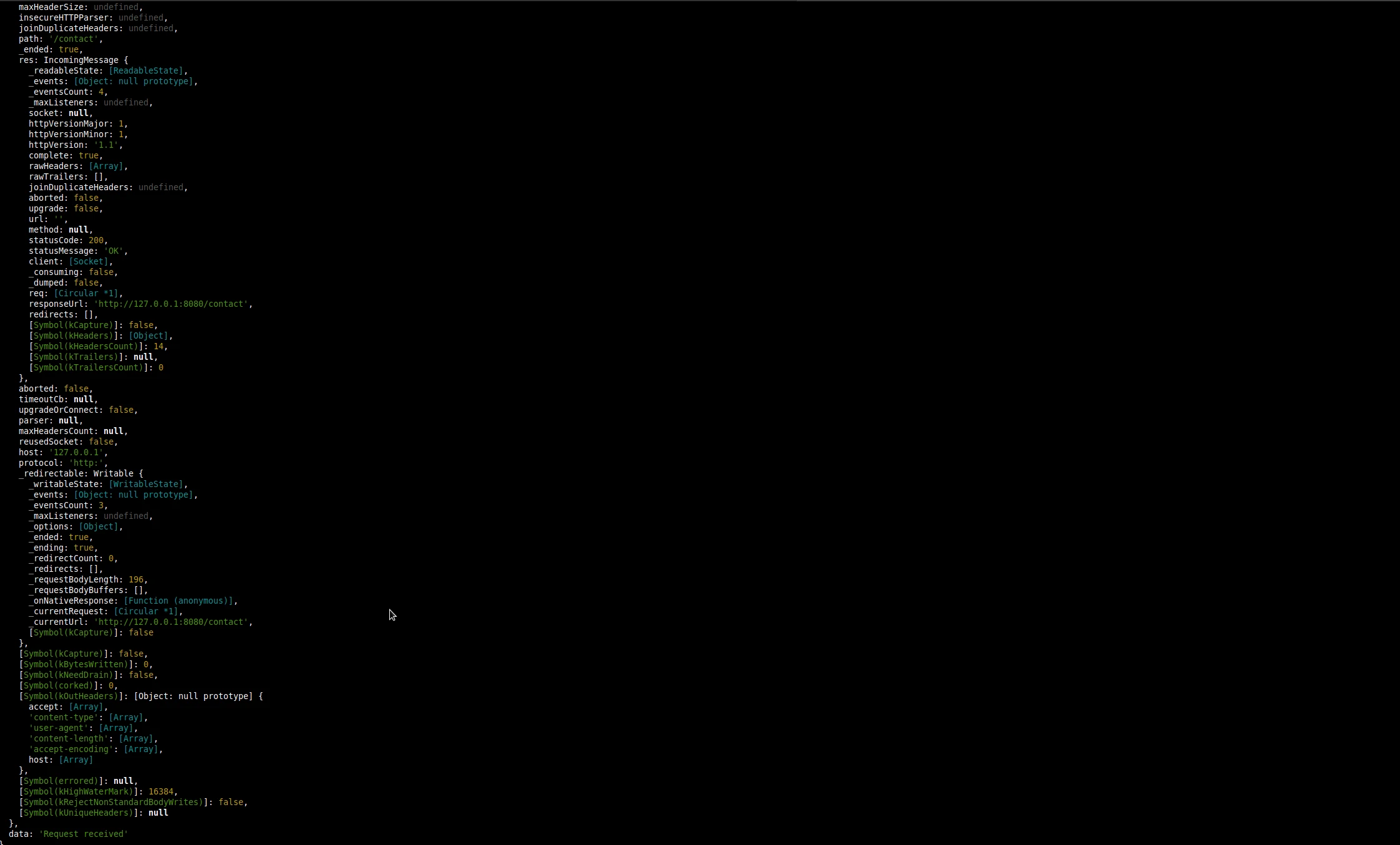